前回の記事の内容に、リレーションの実装を追加します。前回の記事をご覧になっていない方はそちらを先に御覧ください。
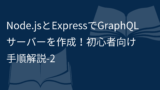
Node.jsとExpressでGraphQLサーバーを作成!初心者向け手順解説②
前回の記事の内容に、スキーマとリゾルバーの定義、クエリとミューテーションの実装を追加します。前回の記事をご覧になっていない方はそちらを先に御覧ください。 const express = require('express'); const {...
この例では、ユーザーとポストのリレーションを扱います。
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
// GraphQLスキーマの定義
const schema = buildSchema(`
type User {
id: Int!
name: String!
posts: [Post]
}
type Post {
id: Int!
title: String!
content: String!
author: User!
}
type Query {
user(id: Int!): User
post(id: Int!): Post
users: [User]
posts: [Post]
}
type Mutation {
createUser(name: String!): User
createPost(title: String!, content: String!, authorId: Int!): Post
}
`);
// サンプルデータ
const users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 3, name: 'Charlie' },
];
const posts = [
{ id: 1, title: 'GraphQL入門', content: 'GraphQLは...', authorId: 1 },
{ id: 2, title: 'REST APIとの違い', content: 'REST APIとは...', authorId: 1 },
{ id: 3, title: 'Expressでの実装', content: 'Expressでの実装方法...', authorId: 2 },
];
// リゾルバ関数の定義
const root = {
user: ({ id }) => users.find(user => user.id === id),
post: ({ id }) => posts.find(post => post.id === id),
users: () => users,
posts: () => posts,
createUser: ({ name }) => {
const newUser = { id: users.length + 1, name };
users.push(newUser);
return newUser;
},
createPost: ({ title, content, authorId }) => {
const newPost = { id: posts.length + 1, title, content, authorId };
posts.push(newPost);
return newPost;
},
};
// UserとPostのリレーション設定
schema.getType('User').getFields().posts.resolve = (user) => posts.filter(post => post.authorId === user.id);
schema.getType('Post').getFields().author.resolve = (post) => users.find(user => user.id === post.authorId);
// Expressサーバーの設定
const app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
// サーバーの起動
app.listen(4000, () => console.log('GraphQLサーバーが http://localhost:4000/graphql で起動しました'));
このソースコードでは、User
と Post
の2つのタイプを定義し、それぞれのリレーションを設定しています。また、ユーザーとポストのクエリとミューテーションも追加しています。http://localhost:4000/graphql で実行できるいくつかのクエリとミューテーションの例を示します。
全てのユーザーを取得するクエリ
{
users {
id
name
posts {
title
}
}
}
全てのポストを取得するクエリ
{
posts {
id
title
content
author {
name
}
}
}
特定のユーザーを取得するクエリ
{
user(id: 1) {
id
name
posts {
title
}
}
}
特定のポストを取得するクエリ
{
post(id: 1) {
id
title
content
author {
name
}
}
}
新しいユーザーを作成するミューテーション
mutation {
createUser(name: "Dave") {
id
name
}
}
新しいポストを作成するミューテーション
mutation {
createPost(title: "GraphQLのアドバンテージ", content: "GraphQLのアドバンテージは...", authorId: 1) {
id
title
content
author {
name
}
}
}
これらのクエリとミューテーションを使って、ユーザーとポストのデータを取得したり、新しいデータを作成したりできます。この例をベースに、更に複雑なデータ構造やリゾルバを追加して、独自のGraphQLサーバーを構築してみてください。